

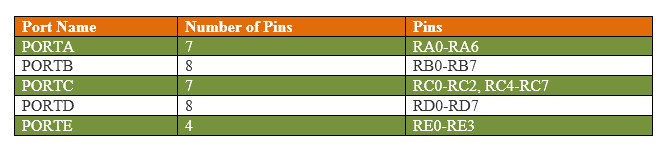
TRISX (TRI STATE)
PORTX
LATX
/* Name : main.c * Purpose : Source code for LED Interfacing with PIC18F4550. * Author : Gemicates * Date : 2017-06-10 * Website : www.gemicates.org * Revision : None */ #include <htc.h> // Header file for PIC18F4550 #define _XTAL_FREQ 12000000 // 12MHZ #define LED PORTB // PORTB as Output #define LED9 PORTCbits.RC0 // PORTC as Output #define LED10 PORTDbits.RD0 // PORTD as Output #define SW PORTDbits.RD1 // PORTD as Output /**********CONFIGURATION BITS SETTING**********/ #pragma config FOSC = HSPLL_HS // Using 20 MHz crystal with PLL #pragma config PLLDIV = 5 // Divide by 5 to provide the 96 MHz PLL with 4 MHz input #pragma config PBADEN = OFF #pragma config CPUDIV = OSC1_PLL2 // Divide 96 MHz PLL output by 2 to get 48 MHz system clock #pragma config FCMEN = OFF // Disable Fail-Safe Clock Monitor #pragma config IESO = OFF // Disable Oscillator Switchover mode #pragma config PWRT = OFF // Disable Power-up timer #pragma config BOR = OFF // Disable Brown-out reset #pragma config WDT = OFF // Disable Watchdog timer #pragma config MCLRE = ON // Enable MCLR Enable #pragma config LVP = OFF // Disable low voltage ICSP #pragma config ICPRT = OFF // Disable dedicated programming port (only on 44-pin devices) #pragma config CP0 = OFF // Disable Code Protection Bit /************************************************/ void main() { TRISB = 0x00; // PORTB as Output TRISC = 0xfe; // RC0 as Output TRISD = 0xfe; // RD0 as Output while(1) // Loop executed infinite times { if(SW==0) // LED turn OFF using Push Button { LED10 = 1; // LED OFF Condition } if (SW==1) // LED turn ON using Push Button { LED10 = 0; // LED ON Condition } LED = 0xff; // LED OFF Condition __delay_ms(50); // delay of 50ms LED = 0x00; // LED ON Condition __delay_ms(50); // delay of 50ms LED9 = 0; // LED ON Condition __delay_ms(50); // delay of 50ms LED9 = 1; // LED OFF Condition __delay_ms(50); // delay of 50ms } }